Unit Testing ConnectRPC Servers
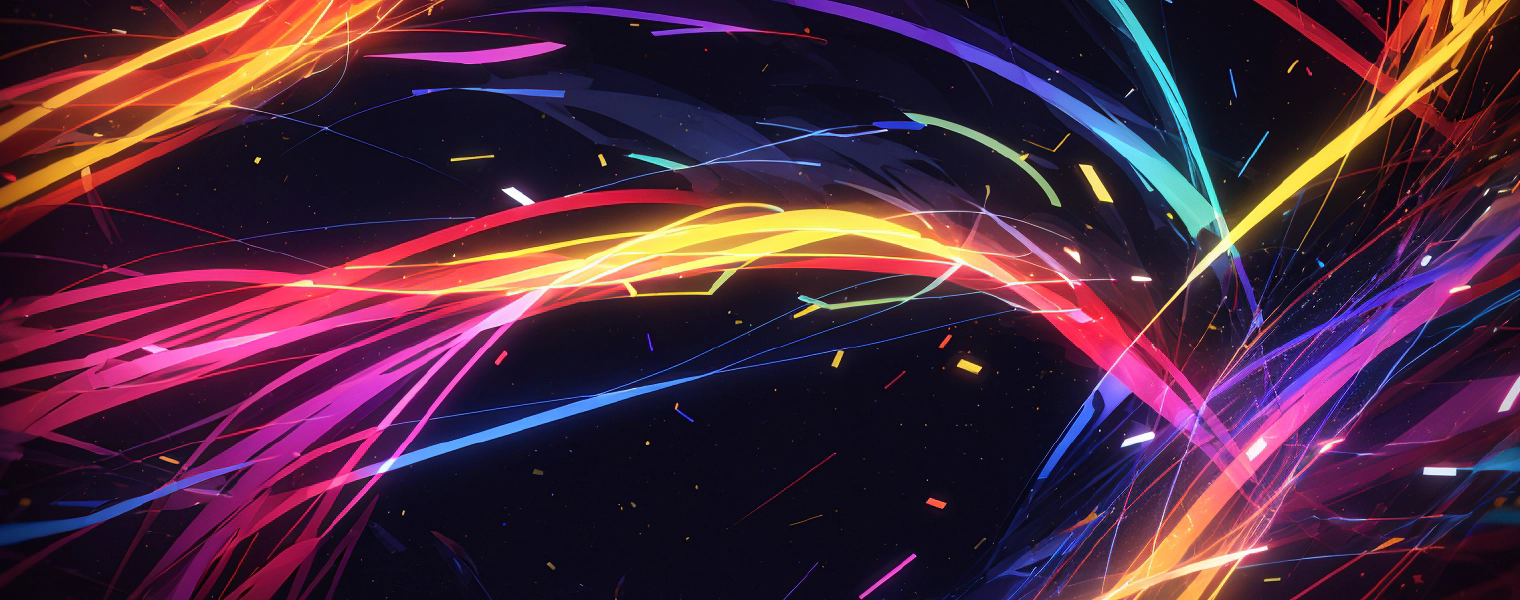
If you’ve embarked on the journey of building efficient and scalable RPC systems with ConnectRPC, you might be pondering the best way to ensure the reliability and correctness of your services. Unit testing is the obvious tool for this, providing a safety net that catches bugs early and empowers you to refactor code fearlessly. In the ConnectRPC world, unit testing can be daunting due to its integration with Protocol Buffers and the client-server architecture. In this guide, we’ll unravel the mysteries of unit testing ConnectRPC services, while arming you with practical examples and advanced techniques to fortify your codebase.
First off, the full source code can be found on github. If it helps, feel free to download, run, and modify as you see fit!
Why Unit Test?
Before we dive in, let’s address the “why.” Unit testing your ConnectRPC servers brings a multitude of benefits:
- Isolation: Focus on testing individual components in isolation, making it easier to pinpoint and fix issues.
- Speed: Unit tests execute quickly, providing fast feedback during development.
- Refactoring Confidence: When you have solid unit tests, you can refactor your code with confidence, knowing that the tests will catch any unintended consequences.
- Documentation: Well-written unit tests can serve as living documentation, illustrating how your code is meant to be used.
- Bug Prevention: A good suite of unit tests can help you catch bugs early on, before they become harder and more expensive to fix.
Testing Strategies with ConnectRPC
ConnectRPC, built upon the Protocol Buffers ecosystem, offers a couple of primary approaches to unit testing:
- Direct Service Testing: This is ideal for unit testing but it’s not always possible. You directly call the methods of your service implementation (typically a struct in Go), bypassing any client and server networking.
- Server Testing: This approach creates an actual ConnectRPC server with
net/http/httptest
. It’s helpful when you want to test the interactions between your client and server code but is usually “overkill” unless you’re wanting to test interceptors or HTTP middleware.
Hands-On: Our example service
Here is the protobuf file that we’re using for our example:
syntax = "proto3";
package greet.v1;
option go_package = "example/gen/greet/v1;greetv1";
message GreetRequest {
string name = 1;
}
message GreetResponse {
string greeting = 1;
}
service GreetService {
rpc Greet(GreetRequest) returns (GreetResponse) {}
}
And here is the resulting server implementation:
type greeterService struct{}
var _ greetv1connect.GreetServiceHandler = (*greeterService)(nil)
func (g *greeterService) Greet(ctx context.Context, req *connect.Request[greetv1.GreetRequest]) (*connect.Response[greetv1.GreetResponse], error) {
if req.Msg.Name == "" {
return nil, errors.New("missing name")
}
// Simulate some network call that takes 10ms
select {
case <-ctx.Done():
return nil, ctx.Err()
case <-time.After(10 * time.Millisecond):
}
return connect.NewResponse(&greetv1.GreetResponse{Greeting: "Hello, " + req.Msg.Name}), nil
}
Here we defined our greetv1connect.GreetServiceHandler
implementation. It implements the Greet
method defined in the protobuf file alove. Since this is for demonstration purposes, all we do is check to see if the given name
is empty, sleep for 10 milliseconds to simulate a network call and returns the greeting as "Hello, {name}"
.
A keep observer might notice that this file contains our first “test”. The line var _ greetv1connect.GreetServiceHandler = (*greeterService)(nil)
is a way of doing a type assertion in Go. It ensures that your greeterService
struct correctly implements the GreeterService
interface defined by the protobuf file above. This relies on a trick of the Go syntax that will try to bind a variable _
using the greetv1connect.GreetServiceHandler
type. If the given greeterService
pointer doesn’t implement the interface then the compiler should complain about what specific methods are missing and which method signatures don’t match.
Hands-On: Direct Service Testing Example
Let’s write some unit tests for a simple ConnectRPC service:
func TestGreet(t *testing.T) {
service := &greeterService{}
response, err := service.Greet(context.Background(), connect.NewRequest(&greetv1.GreetRequest{Name: "Alice"}))
if err != nil {
t.Fatalf("Greet failed: %v", err)
}
if response.Msg.Greeting != "Hello, Alice" {
t.Errorf("Unexpected greeting: got %q, want %q", response.Msg.Greeting, "Hello, Alice")
}
}
Explanation:
The TestGreet
function creates an instance of your greeterService
and directly calls its Greet
method. We then assert that the response matches our expectations. This is, by far, the simplest method for testing a ConnectRPC service.
Hands-On: Table-Driven Tests with Testify
Now that we wrote a single unit test, the next example will show you how to utilize table tests in order to easily write more test cases. You will see code that looks like this in well-tested Go repositories.
func TestGreetTable(t *testing.T) {
service := &greeterService{}
cancelledCtx, cancel := context.WithCancel(context.Background())
cancel()
testCases := []struct {
name string
ctx context.Context
req *connect.Request[greetv1.GreetRequest]
want *connect.Response[greetv1.GreetResponse]
wantErr string
}{
{
name: "Success",
req: connect.NewRequest(&greetv1.GreetRequest{Name: "Bob"}),
want: connect.NewResponse(&greetv1.GreetResponse{Greeting: "Hello, Bob"}),
wantErr: "",
},
{
name: "Empty Name",
req: connect.NewRequest(&greetv1.GreetRequest{}),
want: nil, // Expecting an error
wantErr: "missing name",
},
{
name: "Context Cancelled",
ctx: cancelledCtx,
req: connect.NewRequest(&greetv1.GreetRequest{Name: "Alice"}),
want: nil,
wantErr: "context canceled",
},
}
for _, tc := range testCases {
t.Run(tc.name, func(t *testing.T) {
ctx := tc.ctx
if ctx == nil {
ctx = context.Background()
}
got, err := service.Greet(ctx, tc.req)
if tc.wantErr != "" {
require.Error(t, err)
assert.Contains(t, err.Error(), tc.wantErr)
} else {
require.NoError(t, err)
assert.Equal(t, tc.want, got)
}
})
}
}
Explanation:
- Table Setup: A
testCases
slice defines scenarios with varying inputs (req
), expected outputs (want
), and potential errors (wantErr
). - Context Cancellation: The test case “Context Cancelled” simulates a cancelled context by creating a context with
context.WithCancel
and immediately callingcancel()
. - Testify Assertions: The
require
package is used for assertions that should stop the test if they fail (e.g., requiring an error). Theassert
package is used for assertions that are not critical for continuing the test. Typically, errors during test setup userequire
and assertions on the results of the test useassert
.
Hands-On: Server Testing Example
Here’s how you can test the same service using net/http/httptest
server:
func TestGreetWithServer(t *testing.T) {
mux := http.NewServeMux()
mux.Handle(greetv1connect.NewGreetServiceHandler(&greeterService{}))
server := httptest.NewServer(mux)
t.Cleanup(func() { server.Close() })
client := greetv1connect.NewGreetServiceClient(http.DefaultClient, server.URL)
response, err := client.Greet(context.Background(), connect.NewRequest(&greetv1.GreetRequest{Name: "Alice"}))
if err != nil {
t.Fatalf("Greet failed: %v", err)
}
if response.Msg.Greeting != "Hello, Alice" {
t.Errorf("Unexpected greeting: got %q, want %q", response.Msg.Greeting, "Hello, Alice")
}
}
- Server Setup: We create a ConnectRPC handler and start it with
httptest.NewServer(mux)
. - Client Setup: We create a ConnectRPC client that connects to the server that we just created.
- Test Interaction: We use the client to call the Greet method and assert the response, just like in the direct service testing example.
Conclusion: Test with Confidence
In this guide, we’ve explored the “why” and “how” of unit testing your ConnectRPC services. By embracing unit testing as a core part of your development workflow, you’ll create more robust, reliable, and maintainable RPC systems. Remember, effective testing isn’t just about fixing bugs – it’s about building confidence in your codebase and enabling you to iterate and evolve your services with ease.
The full source code can be found on github.
Next Steps:
- Go Beyond the Basics: Explore more advanced testing techniques, such as mocking dependencies for more complex scenarios.
- Integrate with Your CI/CD: Automate your unit tests to run as part of your continuous integration and continuous delivery (CI/CD) pipeline for immediate feedback on code changes.
- Share Your Knowledge: Help the ConnectRPC community grow by sharing your own testing strategies and experiences!
Ready to put your newfound knowledge into action? Start writing those unit tests and watch your ConnectRPC projects thrive!
Comments
Join the Conversation
Leave a question or comment for future readers who might have the same questions.